It will take about 5 minutes to finish reading this article.
1. “Special” views in SwiftUI
In SwiftUI, every type that follows the View protocol must define a computational property called Body that represents the body content of that view. The type of this Body property determines the structure and content of the view. Typically, the type of Body is a specific View type.
1 | struct MyView: View { |
However, sometimes we create special views whose type of the Body property may not be a specific View type, but a special placeholder type indicating that the view has no actual content. In this case, we can set the type of Body to Never.
Never is a special type that denotes an impossible value or a result that will never be returned. In this context, setting the type of Body to Never means that this view has no actual content; it exists only as a container or modifier for other views.
The purpose of using Body = Never in these view types is to indicate that they have no actual content or may not show any content depending on certain conditions. This avoids processing these views at the SwiftUI runtime, improving performance and efficiency.
Here we introduce a few SwiftUI in several common such special views, they are not directly used in SwiftUI rendering display with, they are mainly used for layout, placeholder, packaging and other roles, they have a very important role in the development of SwiftUI.
2. Introduction to “Special” views
2.1 VStack, HStack, ZStack
UIKit has an invisible view, UIStackView, which is used for arranging other views. In SwiftUI we can create flexible layouts using three view containers, VStack, HStack, and ZStack, which are container views that help us organize and arrange other views to create a variety of complex interfaces.
VStack: a vertical stack layout container that arranges the contained views vertically from top to bottom. This means the views will be laid out vertically in order. As follows:
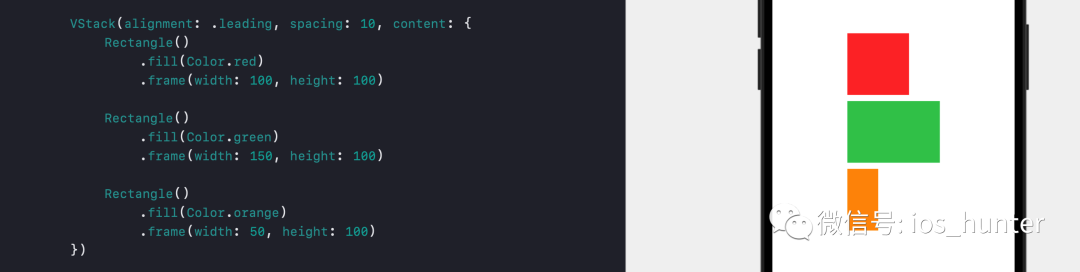
HStack: Horizontal Stack Container View, arranges the contained views horizontally from left to right, and the views will be laid out horizontally in order. As follows:
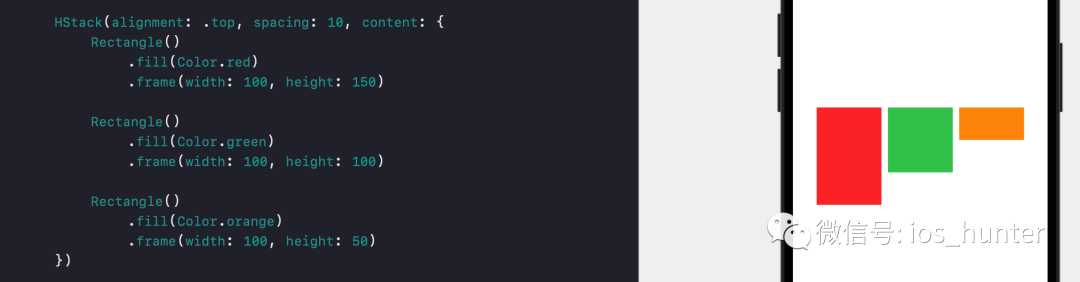
ZStack: cascades container views, cascading the contained views in the order they appear in the ZStack, which means that later views will override earlier ones. As follows:
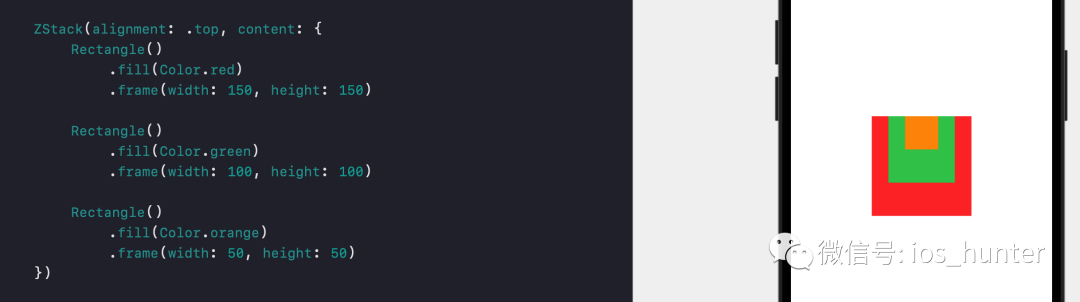
2.2 Spacer()
Spacer is a flexible spatial view that expands along the main axis that contains the Stack layout, or on both x or y axes if it is not included in the stack.Spacer is mostly used in combination with the Stack layout trying to work together. As follows:
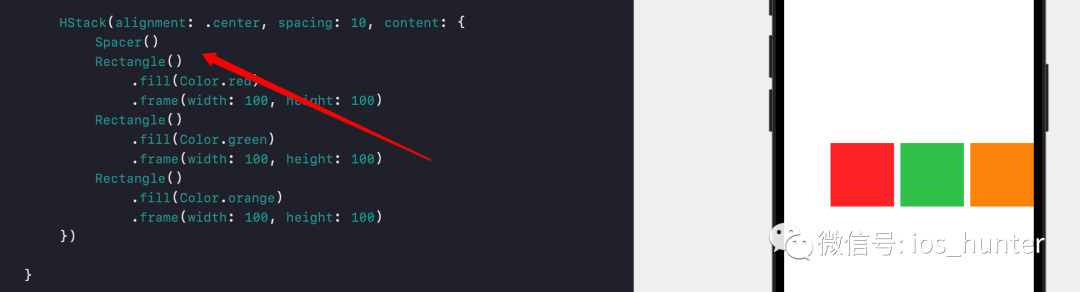
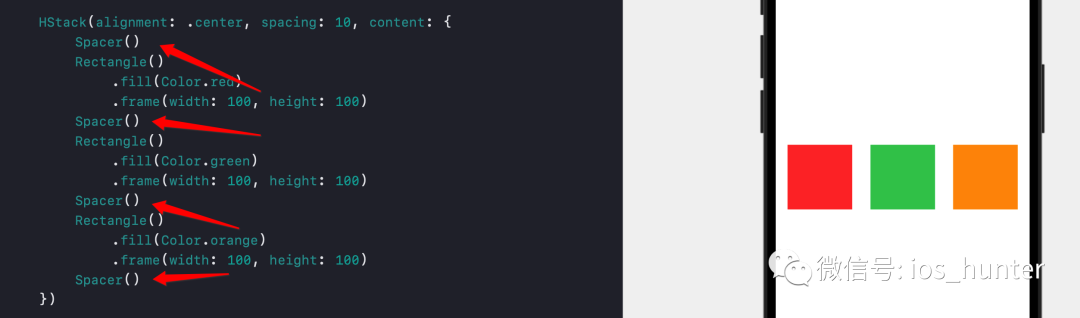
Spacer() will “fill” the space it can occupy.
2.3 Group
Group is a container view in SwiftUI for combining multiple views into a logical whole. It does not apply specific layout or modifications to the views, but is only used to place multiple views in a common container.
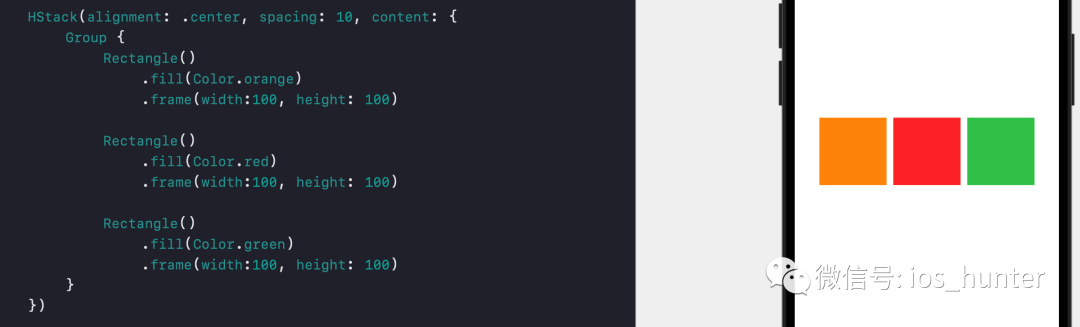
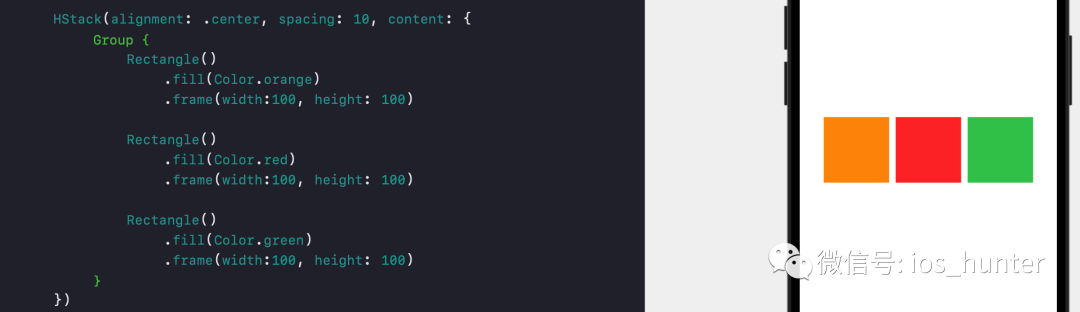
2.4 NavigationView
NavigationView is a container view for building navigation interfaces in SwiftUI. It provides a simple way to manage navigation stacks and navigation links, and provides the user with the ability to navigate interactively.
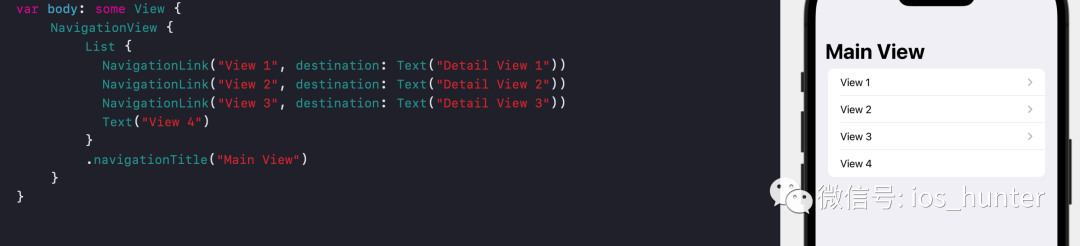
2.5 EmptyView
EmptyView is a special view type in SwiftUI that represents an empty view without any content. It is often used as a placeholder or to hide a view under certain conditions.
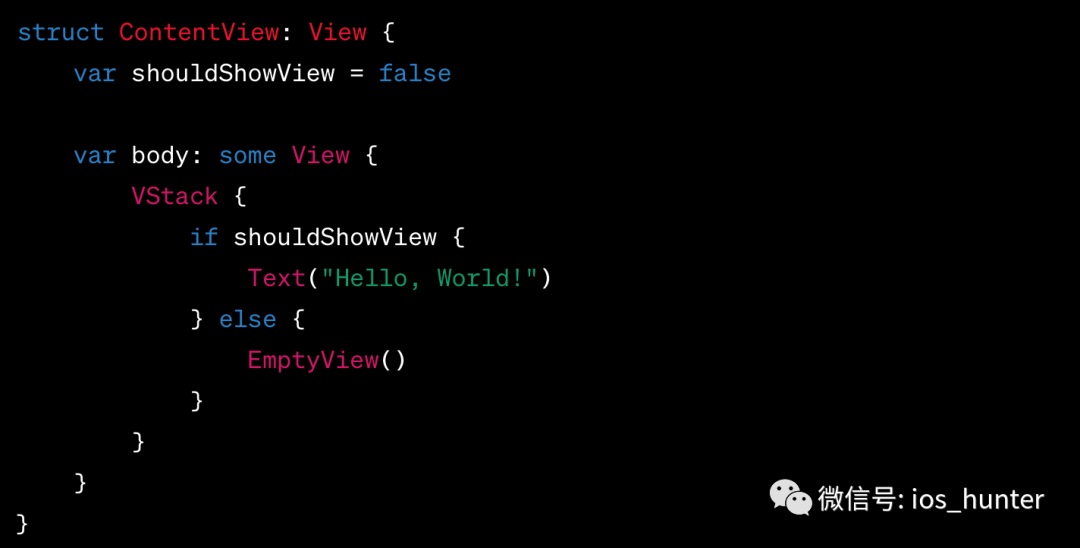
Although EmptyView doesn’t display anything, it’s still a valid view type and can participate in the combination and layout of views. Its presence in SwiftUI gives us the flexibility to control and manage the showing and hiding of views while keeping the code clean and consistent.
2.6 TupleView
TupleView is a view type in SwiftUI for combining multiple views into a tuple view. It allows multiple views to be passed as parameters and combined as a tuple to form a view container.
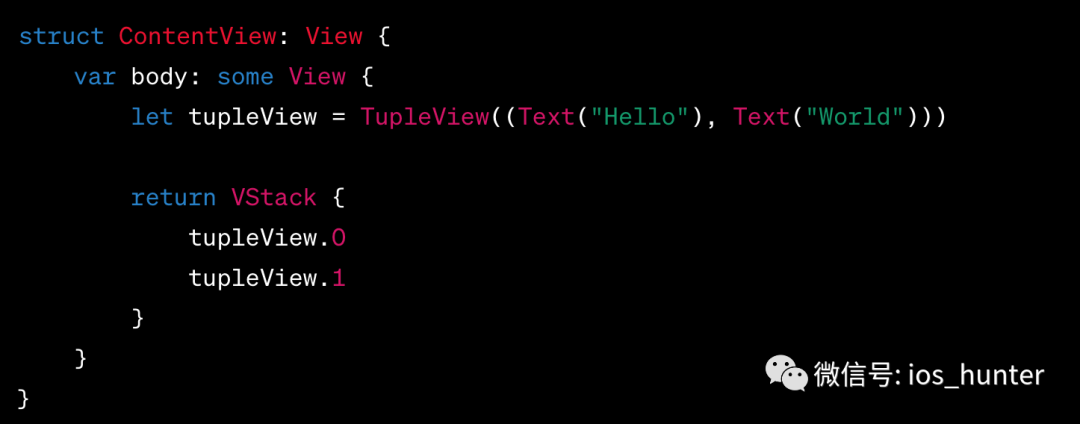
2.7 AnyView
AnyView is a type eraser in SwiftUI that allows to encapsulate any type of view into an opaque type. In SwiftUI, each view has a specific type, but sometimes we need to manipulate views without caring about the specific type, or mix views of different types. This is where you can use AnyView to encapsulate these views and treat them as a unified type. Here is an example showing how to encapsulate and manipulate different types of views using AnyView:
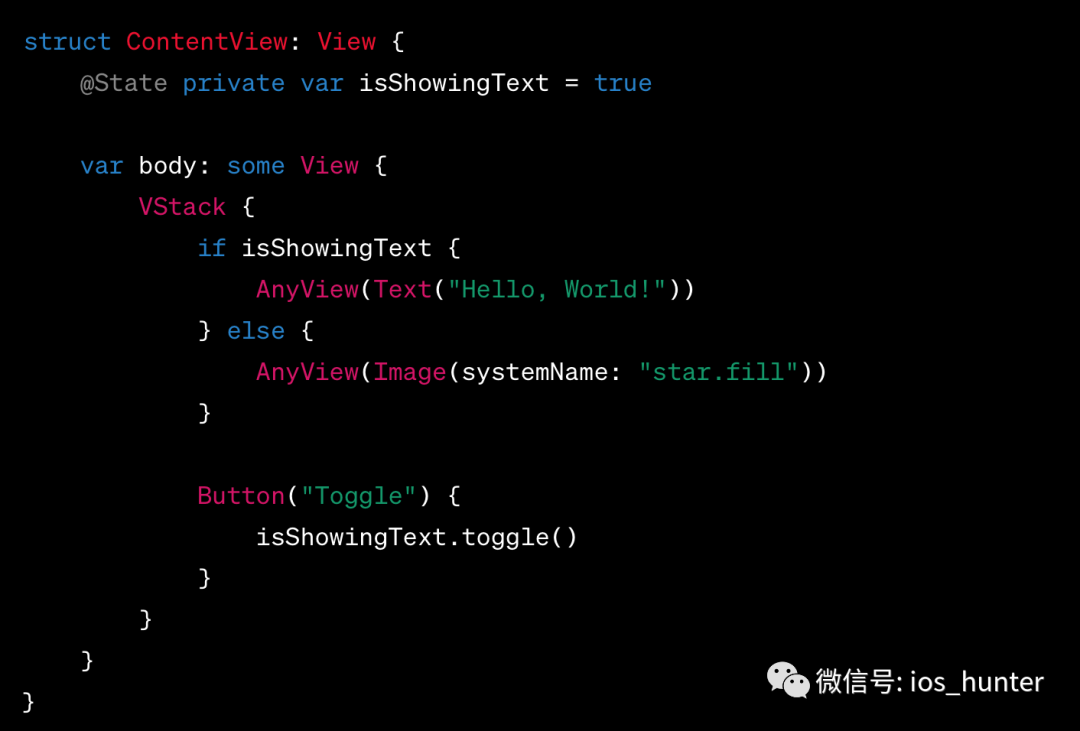
The above list is provided by SwiftUI, of course, we can define some special Views according to our needs.