It will take about 5 minutes to finish reading this article.
1. Combination Operations
A combination operation is the synthesis of multiple Observable streams into a single Observable stream.
1.1 startWith
It is used to insert a specified initial element before the sequence of elements of the Observable sequence, and then emit the elements of the original Observable sequence in turn. In other words, a specific event message is emitted before the event message is emitted. For example, if you emit events 2 , 3 and then I startWith(1), then 1 will be emitted first, then 2 , 3.
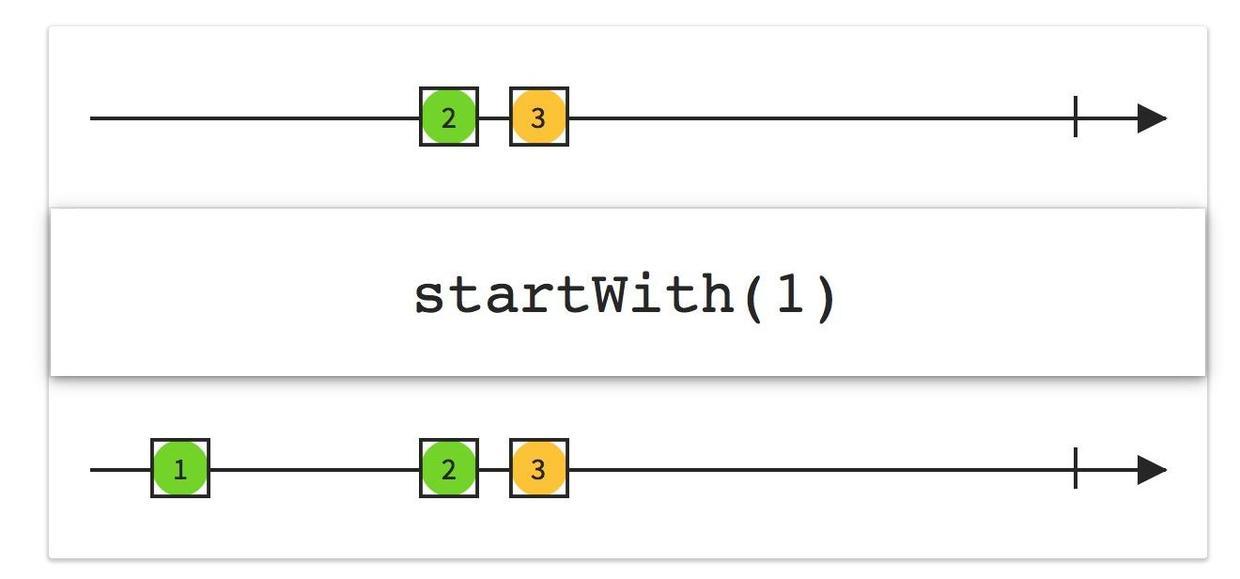
1 | func example15() { |
1.2 merge
Used to merge multiple Observable sequences into a new Observable sequence while maintaining the order in which elements of all original Observable sequences are emitted (corresponding events are emitted according to the timeline).
1 | func example16() { |
1.3 zip
Used to merge the elements of multiple Observable sequences into a new element one by one in order, and emit these new elements in sequence. In other words, the zip operator waits for all participating Observable sequences to emit an element, then merges these elements one-to-one in order into a new element, and then emits the new element.
zip binds up to 8 Observable streams and processes them together. Note that Zip is an event corresponding to an event in another stream.
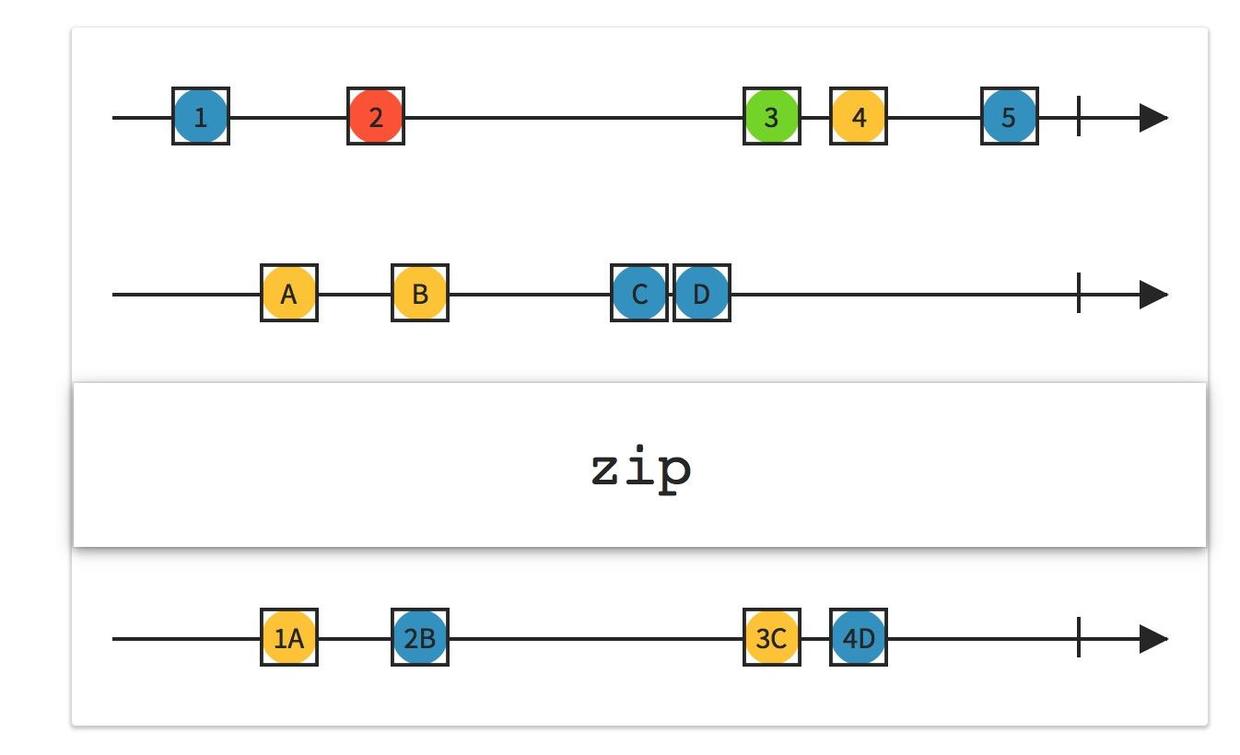
1 | func example17() { |
1.4 combineLatest
Used to combine the latest elements of multiple Observable sequences into a new tuple, and emit this new tuple when any of the original Observable sequences emits a new element. Note: It binds no more than 8 Observable streams. The difference from Zip is that combineLatest is that the event of one stream corresponds to the latest event of another stream. Both events will be the latest events. You can compare the following figure with that of Zip.
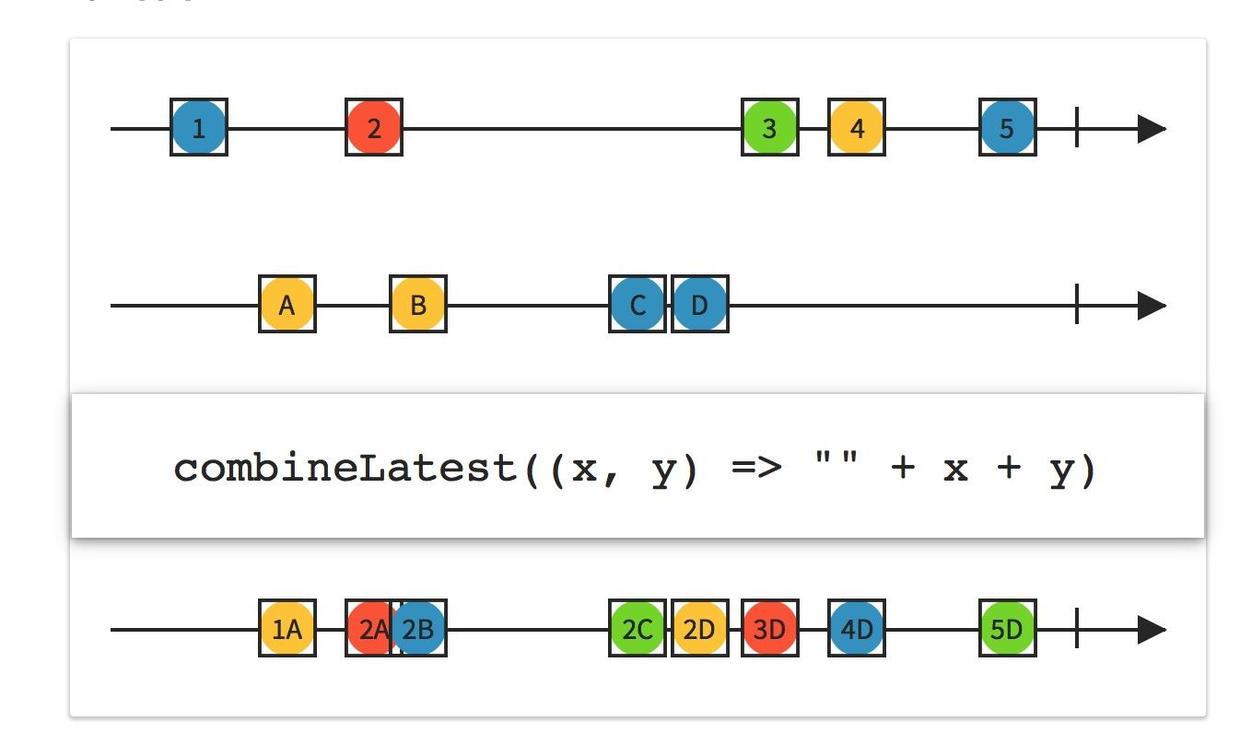
1 |
|
2. Transformation Operations
2.1 map
Used to apply a transformation function to each element in an Observable sequence and emit the transformed elements as a new Observable sequence.
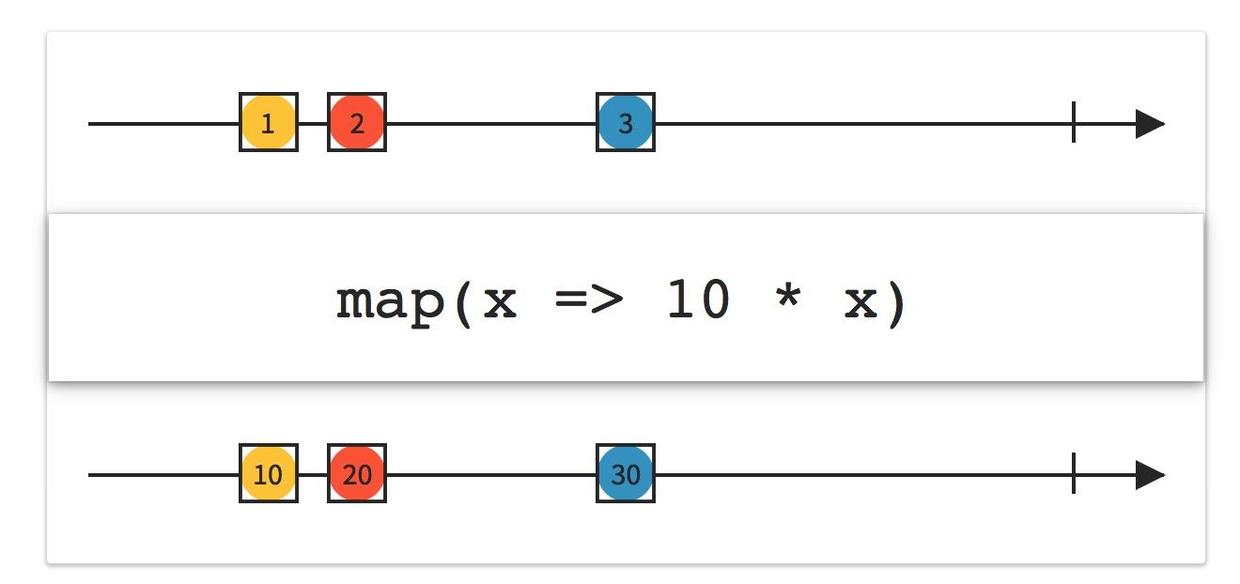
1 | func example19() { |
2.2 flatMap
Used to map each element in the original Observable sequence into a new Observable sequence, then merge these new Observable sequences into a single Observable sequence, and emit elements sequentially in the order in which they were emitted. flatMap has an unpacking action, please see the code analysis.
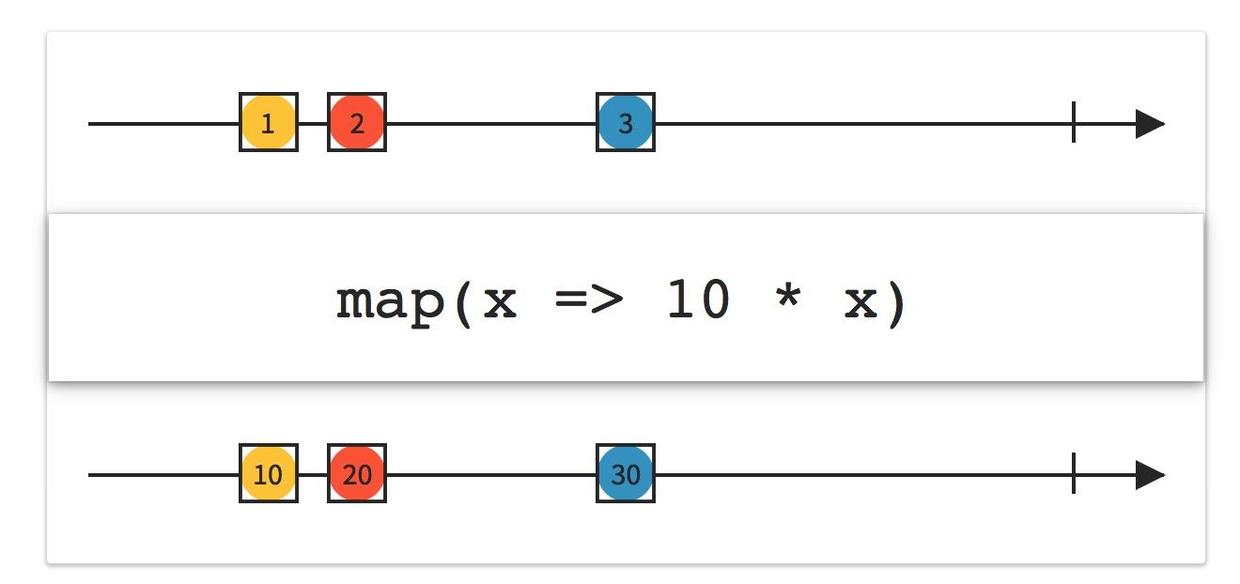
1 | struct Player { |
2.3 flatMapLatest
It is used to map the elements in the original Observable sequence into a new Observable sequence, and only emits the elements of the latest mapped Observable, ignoring the old mapped Observable. Change the above example to flatMapLatest and the result is:
1 | 80 |
2.4 scan
Used to process the elements of an observable sequence one by one according to the specified accumulation rules and generate a new observable sequence that emits the accumulated results of each step.
1 | func example21() { |
3. Filtering And Constraint Operations
3.1 filter
Used to select elements that meet specified conditions from an Observable sequence, and then emit these elements as a new Observable.
1 | func example22() { |
3.2 distinctUntilChanged
It is used to filter out consecutive repeated elements and emit only those elements that are not identical to the previous element. This operation is similar to RAC.
1 | func example23() { |
3.3 elementAt
Used to obtain the element at the specified index position from an Observable sequence and emit it as a new Observable. This operator takes an index value as a parameter and returns a new Observable that emits only the element at the specified index in the original sequence and then completes immediately.
1 | func example24() { |
3.4 single
Used to get a single element from an Observable sequence and emit it as a new Observable. This operator is typically used to ensure that the Observable emits only one element, and it will generate an error event if the Observable emits multiple elements or no elements.
1 | func example25() { |
3.5 take
Used to take a specified number of elements from an Observable sequence and emit them as a new Observable. This operator accepts an integer parameter, indicating the number of elements to be removed.
1 |
|
3.6 takeLast
This operator accepts an integer parameter indicating the number of last elements to be obtained, i.e. it only handles the last few event signals.
1 | func example27() { |
3.7 takeWhile
Used to obtain elements from an Observable sequence based on a certain condition until the condition is no longer met, and emit these elements as a new Observable. This operator accepts a closure argument that returns a Boolean value indicating whether the condition is met.
1 | func example28() { |
3.8 takeUntil
Used to get elements from an Observable sequence if the specified conditions are met, and emit the elements as a new Observable until another specified Observable emits elements or completes.
1 | func example29() { |
3.9 skip
Used to skip a specified number of elements from an Observable sequence and emit the remaining elements as a new Observable.
1 | func example30() { |
3.10 skipWhile
Used to skip elements in an Observable sequence if specified conditions are met, and then emit the remaining elements as a new Observable. This operator accepts a closure argument that returns a Boolean value indicating whether the current element should be skipped.
1 |
|
3.11 skipUntil
Used to skip elements in an original Observable before waiting for another Observable to emit elements. Specifically, skipUntil accepts an Observable as a parameter. When this parameter Observable emits elements, skipUntil will start emitting elements in the original Observable.
1 |
|
4. Math Operations
4.1 toArray
It is used to collect all the elements in an Observable sequence into an array and emit the array as a single-element Observable. In other words, it converts a sequence of multiple elements into an array containing all the elements and emits that array.
1 | func example33() { |
4.2 reduce
It is used to accumulate (reduce) elements in an Observable sequence according to specified rules, and emit the final result as a new Observable.
1 |
|
4.3 Concat
concat will combine multiple sequences into one sequence, and the event of the next sequence will not start until the previous sequence emits the completed event.
Before the first sequence is completed, events emitted by the second sequence will be ignored, but the last event emitted by the second sequence before completion will be received.
1 | func example35() { |
5. Connectable Operations
Connectable Operators are a special class of operators that are used to control situations where multiple observers share the same underlying data source. These operators can convert a normal Observable into a connectable Observable, and then use the connect() method to allow multiple observers to subscribe to this connectable Observable so that they share the same data. Here are some common connectivity operations:
5.1 publish
Convert a normal sequence into a connectable sequence, and a normal Observable into a connectable Observable. It does not start emitting elements immediately, but waits for the connect() method to be called.
1 | let disposeBag = DisposeBag() |
5.2 replay
Converts a normal Observable to a connectable Observable and caches the elements it emits so that subsequent observers can obtain previously emitted elements.
1 | let disposeBag = DisposeBag() |
5.3 multicast
Convert a normal Observable to a joinable Observable. Send it through the characteristic subject, such as PublishSubject, or replaySubject, behaviorSubject, etc. Different Subjects will have different results. Use the connect() method to start emitting elements.
1 | let disposeBag = DisposeBag() |
6. Error Handling
6.1 catchErrorJustReturn
Used to replace the error’s Observable sequence with a predefined default value when an error is encountered, and then continue subscribing to the original Observable. It can be used to handle errors without interrupting the flow of the entire Observable and provide a default value to replace the erroneous element. To put it simply, when encountering an error event, return a default value and end.
1 | let disposeBag = DisposeBag() |
6.2 catchError
Operator for handling errors, which allows you to perform custom error handling logic when an error is encountered, that is, capture the error for processing and return a new Observable to continue subscribing.
1 | let disposeBag = DisposeBag() |
6.3 retry
Used to try to resubscribe to the original Observable when an error is encountered to continue receiving elements. If an error occurs, the retry operator resubscribes to the original Observable and continues to emit elements until the maximum number of attempts is reached or no more errors occur. Especially commonly used when network requests fail.
1 | let disposeBag = DisposeBag() |
7.Debug
7.1 debug
Operator for debugging, which allows you to insert debugging information into the Observable stream to track the Observable’s life cycle, element emission and subscription events, etc. Typically used when developing and debugging RxSwift code to better understand the behavior of Observables.
1 | let disposeBag = DisposeBag() |
7.2 RxSwift.Resources.total
Property used to get the current RxSwift resource count. It is used to monitor the number of resources created and released by RxSwift in the current application. These resources include Observable, Disposable, Operators, etc. Resource counts can help you detect potential resource leak issues or unreasonable resource usage.
1 | let disposeBag = DisposeBag() |
Reference
[1] https://github.com/ReactiveX/RxSwift
[2] https://www.jianshu.com/p/f4ad780cc7a2
[3] https://www.jianshu.com/p/d35a99815806
[4] https://zhuanlan.zhihu.com/p/25939562